サンプルコードに助けられながらもちょっとしたアプリを作成した。
サンプルコードを初めて読んだときは全く意味分からなかったけど
時間が経つにつれてだんだん理解できてきた。
Twiter4jのダウンロード
Twitter4j は Twitter API の Java ラッパだそうです。
javaでTwitter関係のアプリ作ろうとしたらこれが良いらしいので、今回はTwitter4j を使用した。
http://twitter4j.org/ja/index.html
作成したクラス
サンプルコードはcodeZinさんでお世話になりました。http://codezine.jp/article/detail/4409
作成したクラスは3つです。
3つを晒したいと思います(ほぼサンプルw)。
LoginDialog.java
import twitter4j.*; import javax.swing.*; import java.awt.event.ActionListener; import java.awt.event.ActionEvent; import java.awt.*; class LoginDialog extends JDialog implements ActionListener{ JLabel labelName; JLabel labelPass; JTextField textName; JPasswordField passField; JButton okButton; JButton cancelButton; JDialog dialog; public LoginDialog() { JPanel panelOne = new JPanel(); labelName = new JLabel("Name"); textName = new JTextField(15); panelOne.add(labelName); panelOne.add(textName); JPanel panelTwo = new JPanel(); labelPass = new JLabel("Password"); passField = new JPasswordField(15); panelTwo.add(labelPass); panelTwo.add(passField); passField.addActionListener(this); JPanel panelThree = new JPanel(); okButton = new JButton("OK"); cancelButton = new JButton("Cancel"); okButton.addActionListener(this); cancelButton.addActionListener(this); panelThree.add(okButton); panelThree.add(cancelButton); dialog = new JDialog(); dialog.setResizable(false); dialog.getContentPane().add(panelOne); dialog.getContentPane().add(panelTwo); dialog.getContentPane().add(panelThree); dialog.setTitle("Login"); dialog.getContentPane().setLayout(new FlowLayout()); dialog.setSize(350, 150); dialog.setLocationRelativeTo(null); // 画面の中央に配置 dialog.setModal(true); dialog.setVisible(true); } public void actionPerformed(ActionEvent e) { if (e.getSource() == okButton) { dialog.dispose(); } else if (e.getSource() == passField) { dialog.dispose(); } else if (e.getSource() == cancelButton) { System.exit(0); } } public String getUserName() { return textName.getText(); } public String getPassword() { return String.valueOf(passField.getPassword()); } }
TimeLine.java
import twitter4j.*; import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.text.SimpleDateFormat; import java.util.Date; public class TimeLine implements ActionListener { Twitter twitter; JPanel timeLinePanel; JPanel updatePanel; JTextField updateField; JButton updateButton; public TimeLine(Twitter twitter) throws TwitterException{ this.twitter = twitter; timeLinePanel = new JPanel(); updateField = new JTextField(); updatePanel = new JPanel(new FlowLayout()); updatePanel.setSize(600, 30); updatePanel.setPreferredSize(new Dimension(600, 30)); updatePanel.setMaximumSize(new Dimension(600, 30)); updateButton = new JButton("Update"); updateButton.setSize(90, 20); updateButton.setPreferredSize(new Dimension(90, 20)); updateButton.setMaximumSize(new Dimension(90, 20)); updateButton.addActionListener(this); updateField = new JTextField(); updateField.setSize(400, 20); updateField.setPreferredSize(new Dimension(400, 20)); updateField.setMaximumSize(new Dimension(400, 20)); updatePanel.add(updateField); updatePanel.add(updateButton); timeLinePanel.add(updatePanel); updateTimePanel(); } private void updateTimePanel() throws TwitterException { java.util.List<Status> statusList = twitter.getFriendsTimeline(); String statusArr[] = new String[statusList.size()]; timeLinePanel.setLayout(new BoxLayout(timeLinePanel, BoxLayout.Y_AXIS)); for (int i = 0; i < statusList.size(); i++) { Date tweetDate = statusList.get(i).getCreatedAt(); SimpleDateFormat formatter = new SimpleDateFormat("dd-MMM-yy HH:mm"); statusArr[i] = statusList.get(i).getUser().getName() + statusList.get(i).getText() + "-" + formatter.format(tweetDate); } JList statusJList = new JList(statusArr); statusJList.setFixedCellHeight(20); JScrollPane scrollPane = new JScrollPane(statusJList, JScrollPane.VERTICAL_SCROLLBAR_ALWAYS, // 垂直バー JScrollPane.HORIZONTAL_SCROLLBAR_AS_NEEDED); timeLinePanel.add(scrollPane); } public JPanel getTimeLinePanel() { return timeLinePanel; } @Override public void actionPerformed(ActionEvent e) { try { twitter.updateStatus(updateField.getText()); updateTimePanel(); timeLinePanel.remove(1); timeLinePanel.updateUI(); updateField.setText(""); } catch (TwitterException exception) { JOptionPane.showMessageDialog(null, "An error has occurred while updating."); exception.printStackTrace(); } } }
Application.java
import twitter4j.*; import javax.swing.*; import java.awt.*; import java.net.MalformedURLException; public class Application extends JFrame{ public Application() throws MalformedURLException, TwitterException { super("regeek Twitter Application"); getContentPane().setLayout(new BoxLayout(getContentPane(), BoxLayout.X_AXIS)); LoginDialog login = new LoginDialog(); String userName = login.getUserName(); String password = login.getPassword(); Twitter twitter = new TwitterFactory().getInstance(userName,password); add((new TimeLine(twitter)).getTimeLinePanel()); } public static void main(String[] args) throws MalformedURLException, TwitterException { Application application = new Application(); application.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); application.setSize(Toolkit.getDefaultToolkit().getScreenSize()); application.setVisible(true); } }
画像
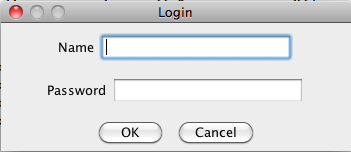
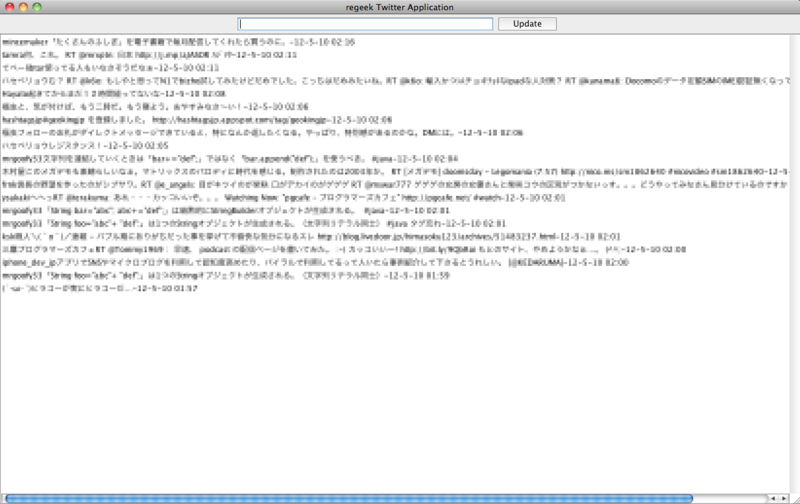
まとめ
今回はTwitterクライアントを作成するという目的でサンプルコードを読んでいたら理解するまでに意外に時間がかかってしまった。まさか丸一日かかるとは思ってもいなく、結構大変だった。
大変だった原因としては、クラスの種類をあまりわかっていなかったということ。
特にTwitter4jのクラスについて・・・
今までのjavaプログラミングで、様々なクラスや、そのクラスからのメソッドをあまり使用していなかったことがわかった。
このコードの理解度はまだ60%程度なので、ドキュメントを見てさらに理解度のパーセンテージを上げたいと思う。
今後はGUIにこだわって改善していきたい。